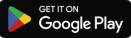
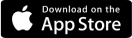
Tega Oke
SHARE THIS POST
The ECMAScript specification (ECMAScript Spec) is a standardized specification developed by Brendan Eich for the Javascript programming language.
This specification ensures that javascript behaves the same across platforms where javascript runs (Browser engines, Node). Software engineers, language designers, and their like often submit proposals to the ECMAScript Spec. These proposals pass through five stages (stages 0 to 4) of development and evaluation by the TC39 (Technical Committee 39), the community responsible for the evolution of ECMAScript, before they are added to the ECMAScript spec.
This article will explore some interesting ECMA Spec proposals that have reached stage 4 and may be added to the next ECMAScript Spec release. Shall we?
If you are familiar with the Lodash groupBy method or the SQL GROUP BY clause, you’d easily understand what this proposal aims to achieve. This proposal seeks to give ECMAScript the ability to combine data into groups.
This groupBy method would be accessible as properties in the Object and Map data structure. For example, say you want to group an array of objects based on the type property; you could do this using the groupBy method.
The above code sample uses the groupBy method to group an array of animals and persons into their respective groups. This would be a wonderful addition to the ECMA Spec as it would make it a little straightforward. This method also has a polyfill available here if you’d like to play around with it
Decorators are functions that allow you to modify or add behavior to classes, functions, or methods without altering the original code. Although Javascript developers have been using this through transpilers like Babel and Typescript, It would be interesting to keep an eye on this proposal as it would provide a standard syntax for decorators to Javascript.
This proposal provides array methods that make changes to arrays without mutating the original array. It returns a copy of the array containing the changes. The change array copy proposal is finished and has been merged to the ECMAScript spec and is available in ES2023.
It provides methods to sort, reverse and change array data without mutating the array these data are contained in but returns a new copy of the array with the change. Here is an example using the toReversed method
This proposal aims to be more consistent and reliable than the current javascript Date object. It also fixes the mutability with the Date object, adds more time zones other than UTC or users’ local time, and has many more exciting features.
Here is an example of Temporal immutability compared to the Date object using a Temporal polypill
The immutability of Temporal helps to prevent unexpected modifications to the date/time data.
A lot of exciting features will be released with Temporal, which would fix issues currently present in Javascript’s Date object. You can check the Temporal documentation for more exciting features.
You can find more proposals in the official ECMAScript proposal repo here.
Thank you for taking time out to learn about the exciting things coming to Javascript. I hope you enjoyed reading this and learned a thing or two.
Back to top